
Obsidian Typing
The most comprehensive tool to customize groups of notes in Obsidian
Obsidian Typing
The most comprehensive tool to customize groups of notes in Obsidian
Configuration as Code
Unleash the Obsidian Typing Language (OTL) for Ultimate Customization:
- Starting Out: Quickly categorize notes with specific folders, icons, and field structures. Experience a more structured and personalized note-taking journey.
- Going Deeper: Dive deep with advanced customizations, utilizing complex note type inheritance and beyond. Customize Obsidian intricately to your workflows and imagination.
import { Hierarchical } from "../hierarchical"
import { Actionable } from "../actionable"
type Issue extends Hierarchical, Actionable {
folder = "typed/issues"
prefix = "I-{serial}"
fields {
tags: List[Tag[dynamic=true]]
due: Date
}
style {
header = fn"""..."""
link = fn"""..."""
css_classes = ["max-width"]
}
hooks {
on_create = fn"""..."""
on_open = fn"""..."""
}
methods {
children = expr"""..."""
}
}
Field Types
Precisely define field types for every note type, ensuring consistent structure across notes. Benefit from an intuitive UI, making both note creation and modifications streamlined and user-friendly.
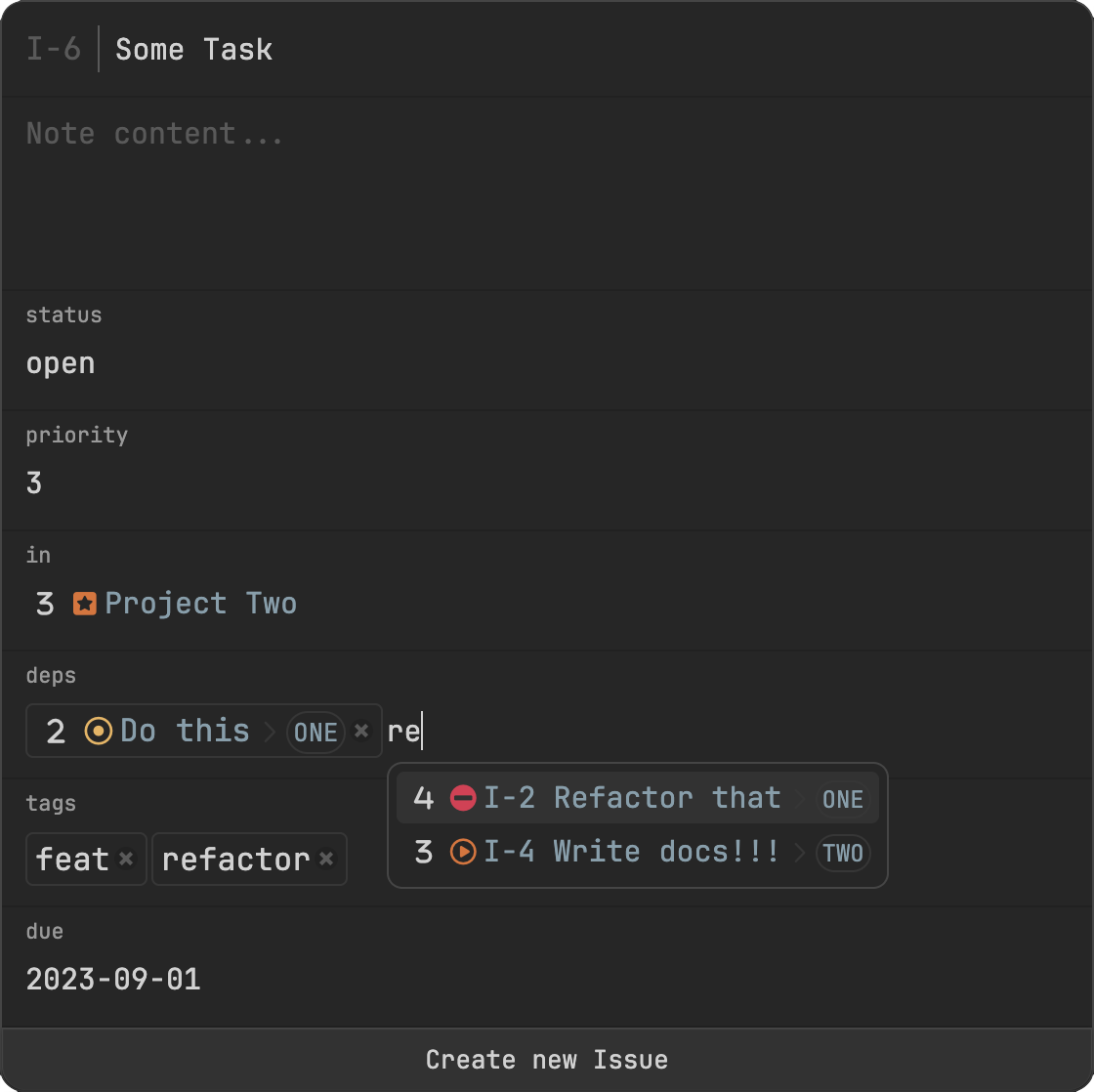
type Issue {
folder = "typed/issues"
icon = "far fa-circle-dot"
prefix = "I-{serial}"
fields {
status: Choice["backlog", "open", "closed"] = "backlog"
priority: Number[min=1, max=5] = 2
in: Note["Project", "Issue"]
deps: List[Note["Issue"]]
tags: List[Tag[dynamic=true]]
due: Date
}
}
Headers & Footers
Enhance your notes using auto-injected React components or Markdown content in headers and footers, avoiding unnecessary template clutter.
type Issue {
style {
header = fn"""
import {Breadcrumb} from "packages/breadcrumb"
import {Wikihead} from "packages/wiki"
return <>
<Breadcrumb note={note} base={"apps/Issues.md"} />
<Wikihead note={note} exclude={["in"]} />
</>
"""
footer = fn"""
import {IssueFooter} from "./footer"
return <IssueFooter note={note} />
"""
}
}
Source Mode
status :: active
priority :: 4
in :: [[PRJ Project One]]
due :: 2023-10-31
deps :: [[I-1 Do this]], [[I-4 Write docs!!!]]
tags :: "refactor",
Subtasks:
- [ ] one
- [ ] two
Preview Mode
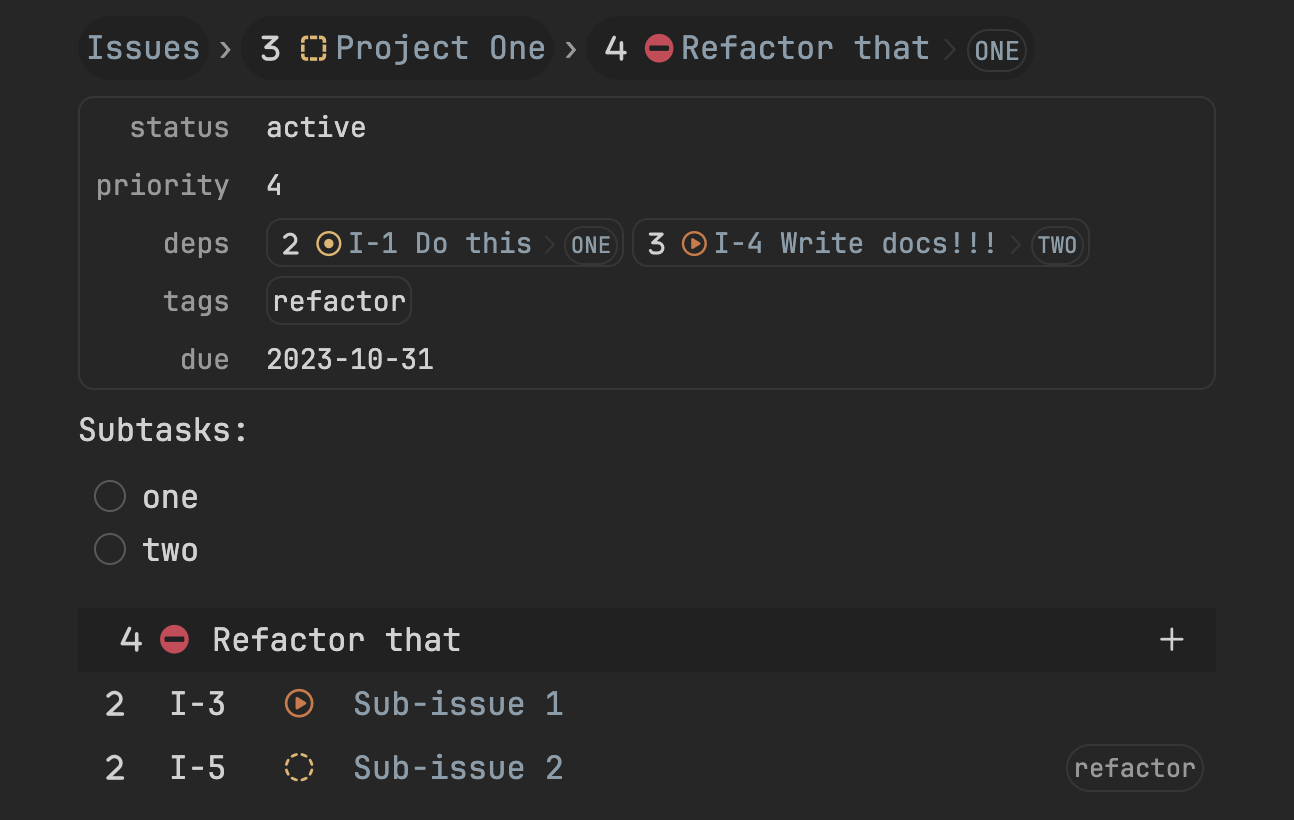
Interactive Links
Replace internal links with dynamic React components. Visualize "Project" statuses or display avatars for "Person" notes seamlessly.
Source Mode
- Discussed [[PRJ Project One]] with [[@ElonMusk]] and [[@JohnDoe]]
Preview Mode

type Person {
style {
link = fn"""
import {Container, Avatar, Icon} from "./utils.tsx"
const abbrev = note.page.name[0] + note.page.surname[0];
const photo = note.methods.photo();
if (photo)
return <Container><Avatar src={src} />{abbrev}</Container>;
}
return <Container><Icon className="far fa-user" />{abbrev}</Container>;
"""
}
methods {
photo = fn"""() => {
const page = note.page
if (!page.photo) return;
const vault = api.app.vault;
const tfile = vault.getAbstractFileByPath(page.photo.path)
if (!tfile) return;
return vault.getResourcePath(tfile)
}"""
}
}
CSS Classes or Code
Bypass individual note styling in Obsidian. Apply CSS classes or inject CSS code across the entire note types.
type A extends B {
style {
css = css"""
& a {
text-decoration: underline
}
"""
css_classes = ["max-width", "cards"]
}
}
Dynamic Prefixes
Craft personalized note naming conventions with versatile prefixes. From serial numbers to compact dates and beyond, tailor your prefixes to your needs.
type Meeting {
prefix = "MTN-{serial}"
}
MTN-1
MTN-2
MTN-3
- ...
type Meeting {
prefix = "MTN-{date_compact}"
}
MTN-M1A9WM
MTN-N6BF3d
MTN-N869mz
- ...
Advanced Scripting & Module System
Effortlessly import OTL types and [J/T]S[X] symbols with intuitive syntax. Rapidly develop your headers, while we watch module changes and refresh your components in real time.
import {A} from "packages/a"
import {B} from "packages/b"
type A extends B {
style {
header = fn"""
import {Breadcrumb} from "./breadcrumb"
return <Breadcrumb note={note} />
"""
}
}
import * as Utils from "packages/utils"
export const Breadcrumb = ({note}) => {
// ...
}
export function do_something() {
return "something"
}
Full-featured OTL Editor
Discover OTL with confidence. Enjoy syntax autocompletion, handy widgets, and real-time linting, making OTL development a breeze.
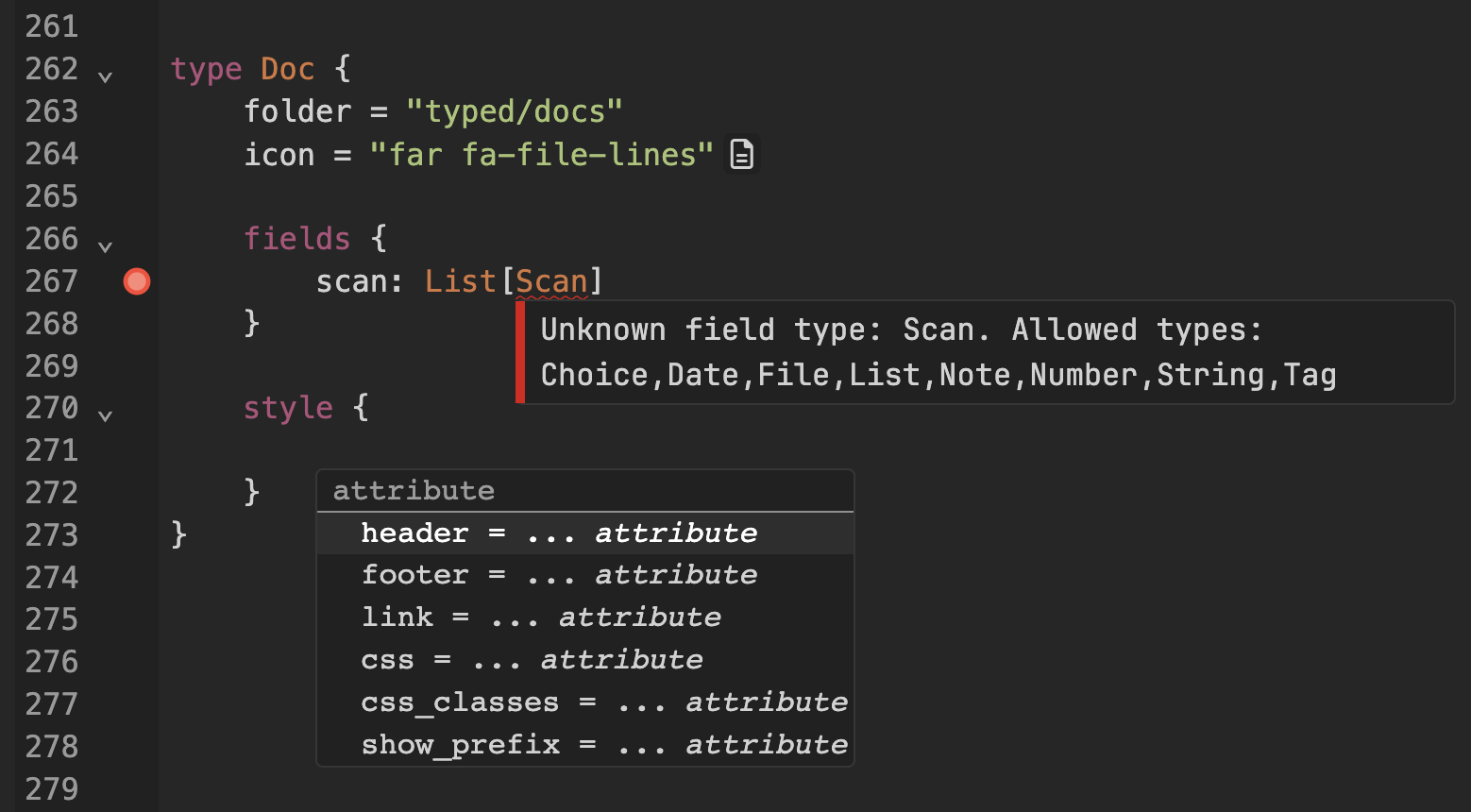
Community Packages
An ecosystem of packages awaits! Create them to share your vision, or install to improve your workflow.
Use packages as easily as:
import { Issue, Project, _IssuesApp } from "konodyuk:issues@1.0"
---
_type: _IssuesApp
---